Among the many exciting features of AngularJS, the ability to navigate smoothly within a webpage using Angular Scroll to Top is gaining popularity. This guide will take a deep dive into this concept and how you can apply it to your web applications.
Understanding the Basics of AngularJS
AngularJS, an open-source web application framework, brings structure to web development, allowing developers to build dynamic, single-page apps quickly and efficiently. It provides built-in directives or reusable components that handle a variety of tasks. One such feature we will be exploring in this guide is the Angular Scroll to Top directive.
Scroll to Top with AngularJS
AngularJS can be used to enhance user navigation within a webpage. Specifically, Angular Scroll to Top allows developers to implement an automatic scroll back to the top of the webpage. This functionality proves essential when dealing with long webpages, improving user experience as they don’t need to manually scroll back to the top.
Here is an example of how you can employ this feature in your AngularJS application:
<div ng-app="myApp">
<p>Top</p>
<div>
<a scroll-to="section1">Go to Section 1</a>
</div>
<div style="padding-top:1000px">...</div>
<div id="section1">
<h2>Section 1</h2>
<a scroll-to="">Back to Top</a>
</div>
</div>
In the example above, the ‘a’ element with the scroll-to attribute creates a clickable link that navigates the user to “section1”. The last ‘a’ element provides a link to scroll back to the top of the page.
Integrating ngScrollTo in Your AngularJS Application
ngScrollTo is a simple AngularJS directive that allows you to implement the scroll-to functionality. It is an attribute directive that listens for click events and scrolls to the target element. Here’s an example:
<div ng-app="app">
<div ng-controller="myCtrl">
<a scroll-to="section1">Section 1</a>
</div>
...
<div id="section1">
<h2>Section 1</h2>
<a scroll-to="">Back to Top</a>
</div>
...
<div id="section1">
<h2>Section 1</h2>
<a scroll-to="section1" offset="60">Section 1 with 60px offset</a>
</div>
</div>
You can even add an offset, which allows a certain space between the section top and the page top when scrolling.
Modifying the Scroll to Top Directive
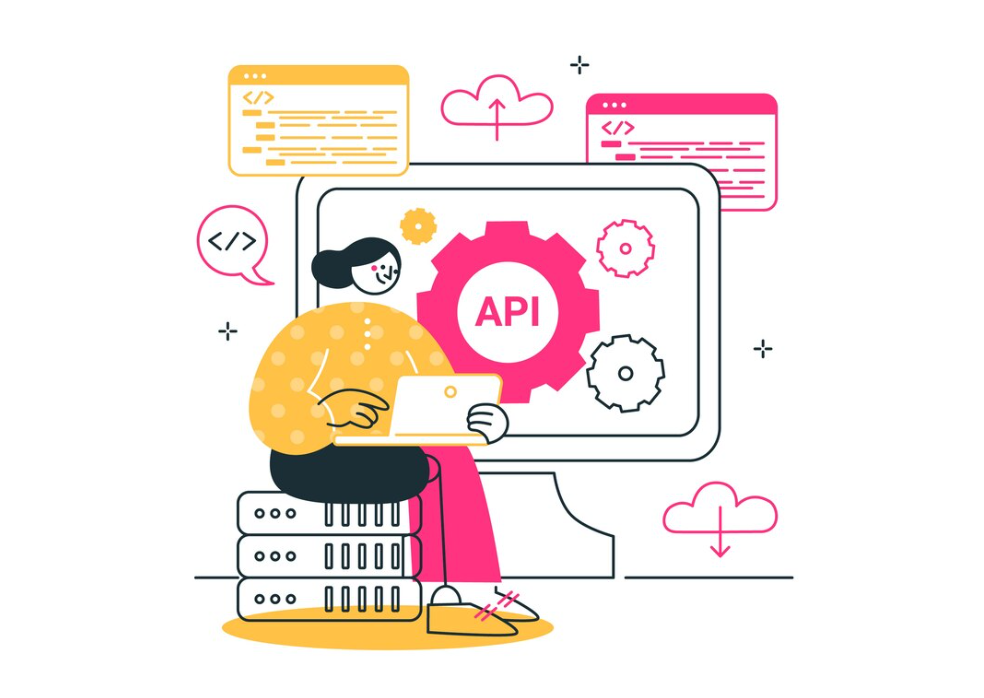
You can alter ngScrollTo to suit the needs of your application. Here’s an example:
angular.module('ngScrollTo', []);
angular.module('ngScrollTo')
.directive('scrollTo', ['ScrollTo', function(ScrollTo) {
return {
restrict : "AC",
compile : function() {
return function(scope, element, attr) {
element.bind("click", function(event) {
ScrollTo.idOrName(attr.scrollTo, attr.offset);
});
}
}
};
}])
The ‘scrollTo’ directive is created, that on being clicked, triggers the ‘idOrName’ method of ‘ScrollTo’ service with provided id or name and offset.
Adjusting Scroll Offset with AngularJS
You can adjust the scroll offset in AngularJS to create space between the document’s top edge and the element’s top edge when scrolling. In the example below, “section1” is being scrolled to with a 60px offset.
<a scroll-to=”section1″ offset=”60″>Section 1 with 60px offset</a>
How ngScrollTo Services Works
ngScrollTo uses a service called “ScrollTo” that handles the scroll function. If no id or name is provided, the page will scroll to the top. It also checks if an element can be found with the id or name attribute. If such an element is found, it scrolls to that element. Here’s an example of how this service is built:
.service('ScrollTo', ['$window', 'ngScrollToOptions', function($window, ngScrollToOptions) {
this.idOrName = function (idOrName, offset, focus) {
var document = $window.document;
//move to top if idOrName is not provided
if(!idOrName) {
$window.scrollTo(0, 0);
}
//check if an element can be found with id attribute
var el = document.getElementById(idOrName);
if(!el) {
el = document.getElementsByName(idOrName);
...
}
...
}
}])
Conclusion
Understanding the Angular Scroll to Top directive can significantly improve the user experience in your AngularJS applications. This guide walked you through the principles of AngularJS and how to integrate ngScrollTo into your application, modify the scroll-to directive, adjust scroll offset, and how the ngScrollTo service works. Armed with this knowledge, you are now ready to enhance the navigation within your AngularJS applications.