As the popularity of web development grows, so does the number of tools available to developers. AngularJS Panels are one such tool, offering a pure AngularJS framework for creating dynamic side panels on your web page. This feature eliminates the need for jQuery, streamlining your web development process.
Installation Process
Getting started with AngularJS Panels is easy.
- Simply download the designated JavaScript and CSS files into your project folder;
- Once that’s done, insert the necessary script and link tags into your HTML page;
- To activate the panels, include a panels container tag within your application.
Panel Configuration
To configure a panel, you need to add a dependency to your application module and configure the panel settings.
- You can set the unique ID, position, dimensions, template URL and controller name for each panel;
- You can also specify callback functions for opening and closing the panel.
Integrating Angular Panels into Your Web Project
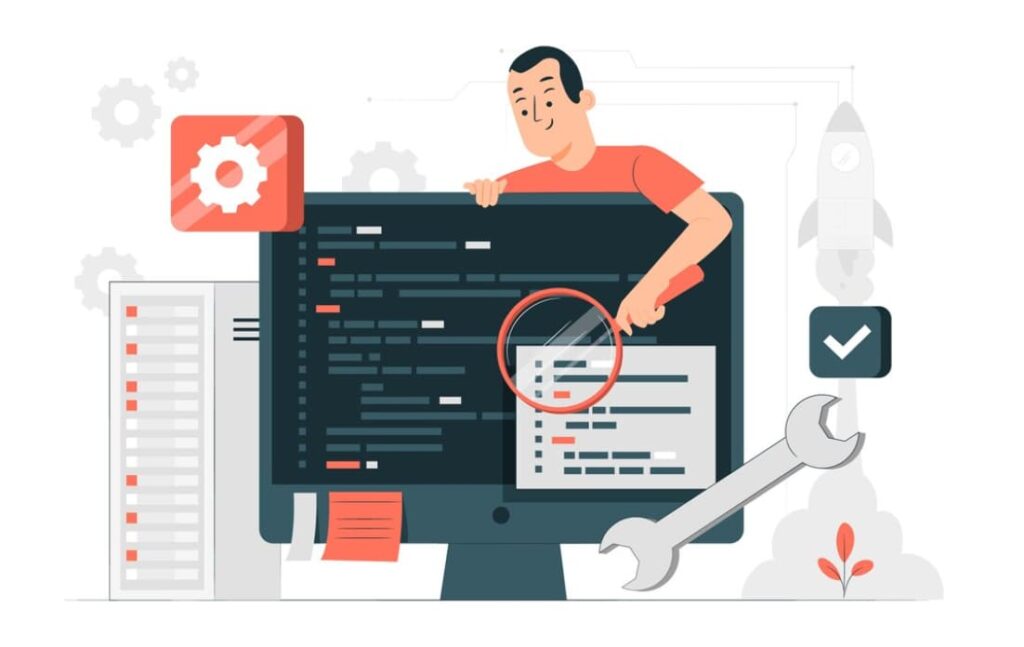
- To incorporate Angular Panels into your project, first, embed the Angular Panels script and stylesheet into your webpage by adding the following tags:
<script type="text/javascript" src="angular.panels.js"></script>
<link rel="stylesheet" type="text/css" href="angular.panels.css">
- Next, insert the panels container tag within your application’s HTML structure:
<div data-panels="true"></div>
- Proceed by adding angular.panels as a dependency to your AngularJS module, and use the configuration block to set up the panels as needed:
var app = angular.module('myApp', ['angular.panels']);
app.config(['panelsProvider', function (panelsProvider) {
panelsProvider
.add({
id: 'testmenu',
position: 'right',
size: '700px',
templateUrl: '../resources/template/testmenu.html',
controller: 'testmenuCtrl'
})
.add({
id: 'testpanel',
position: 'right',
size: '80%',
templateUrl: '../resources/template/testpanel.html',
controller: 'testpanelCtrl',
closeCallbackFunction: 'testpanelClose'
});
}]);
For each panel, you can define the following attributes:
Field | Description |
---|---|
id | A unique identifier for the panel. |
position | Specifies from which edge of the screen the panel will appear (top, right, bottom, or left). |
size | Determines the height or width of the panel. Must include a unit of measurement (e.g., px, em, %). |
templateUrl | The URL path to the panel’s HTML template. |
controller | The name of the controller associated with this panel. |
openCallbackFunction | A function to call when the panel is opened. |
closeCallbackFunction | A function to call when the panel is closed. |
To access the panel, integrate the panels service into your application and invoke the service method as shown below.
var app = angular.module('myApp', ['angular.panels']);
app.config(['panelsProvider', function (panelsProvider) {
panelsProvider
.add({
id: 'testmenu',
position: 'right',
size: '700px',
templateUrl: '../resources/template/testmenu.html',
controller: 'testmenuCtrl'
});
}]);
app.controller('defaultController', function($scope, panels) {
//open testmenu panel
panels.open('testmenu');
});
app.controller('testmenuCtrl', function($scope) {
//left panel controller
});
- That’s it. The complete source code for the demo is provided below.
index.html
<!DOCTYPE html>
<html data-ng-app="angularApplication">
<head lang="ko">
<meta charset="utf-8">
<link href="//rawgit.com/eu81273/angular.panels/master/dist/angular.panels.min.css" rel="stylesheet" type="text/css">
<script src="//cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.14/angular.min.js"></script>
<script src="//rawgit.com/eu81273/angular.panels/master/dist/angular.panels.min.js"></script>
</head>
<body data-ng-controller="defaultController">
<div class="container-fluid content-section">
<div class="container">
<div class="row">
<h2><i class="fa fa-play-circle"></i> Example</h2>
<p>Type any message you want to show on the panel in the input box below, and press the button "LEFT OPEN".</p>
<div class="form-group">
<input class="form-control input-lg" type="text" data-ng-model="message" placeholder="Message for panels..">
</div>
<div class="btn-group btn-group-justified">
<a href="" data-ng-click="leftOpen()" class="btn btn-default">Left Open</a>
<a href="" data-ng-click="rightOpen()" class="btn btn-default">Right Open</a>
<a href="" data-ng-click="topOpen()" class="btn btn-default">Top Open</a>
<a href="" data-ng-click="bottomOpen()" class="btn btn-default">Bottom Open</a>
</div>
</div>
</div>
</div>
<div data-panels="true"></div>
<script>
var app = angular.module('angularApplication', ['angular.panels']);
//add panels
app.config(['panelsProvider', function (panelsProvider) {
panelsProvider
.add({
id: 'test01',
position: 'left',
size: '700px',
templateUrl: '../resources/template/left.html',
controller: 'leftCtrl'
})
.add({
id: 'test02',
position: 'right',
size: '50%',
templateUrl: '../resources/template/right.html',
controller: 'rightCtrl'
})
.add({
id: 'test03',
position: 'top',
size: '20%',
templateUrl: '../resources/template/top.html',
controller: 'topCtrl'
})
.add({
id: 'test04',
position: 'bottom',
size: '80%',
templateUrl: '../resources/template/bottom.html',
controller: 'testpanelCtrl',
closeCallbackFunction: 'bottomClose'
});
}]);
//default controller
app.controller('defaultController', ['$scope', 'panels', function ($scope, panels) {
$scope.leftOpen = function () {
$scope.$broadcast('leftHello', {message : $scope.message});
};
$scope.rightOpen = function () {
$scope.$broadcast('rightHello', {message : $scope.message});
};
$scope.topOpen = function () {
$scope.$broadcast('topHello', {message : $scope.message});
};
$scope.bottomOpen = function () {
$scope.$broadcast('bottomHello', {message : $scope.message});
};
}]);
//left panel controller
app.controller('leftCtrl', ['$scope', 'panels', function ($scope, panels) {
$scope.$on('leftHello', function(event, args) {
$scope.message = args.message;
panels.open("test01");
});
}]);
//right panel controller
app.controller('rightCtrl', ['$scope', 'panels', function ($scope, panels) {
$scope.$on('rightHello', function(event, args) {
$scope.message = args.message;
panels.open("test02");
});
}]);
//top panel controller
app.controller('topCtrl', ['$scope', 'panels', function ($scope, panels) {
$scope.$on('topHello', function(event, args) {
$scope.message = args.message;
panels.open("test03");
});
}]);
//bottom panel controller
app.controller('bottomCtrl', ['$scope', 'panels', function ($scope, panels) {
$scope.$on('bottomHello', function(event, args) {
$scope.message = args.message;
panels.open("test04");
});
//close callback
$scope.bottomClose = function () {
window.alert('Close Callback!!');
};
}]);
</script>
</body>
</html>
left.html
<div class="pane-head">
<button type="button" class="close" data-ng-click="panels.close();">×</button>
</div>
<div class="pane-body">
<div class="row">
<h3>Left Panel</h3>
</div>
<div class="row">{{message}}</div>
</div>
Conclusion
AngularJS Panels offer a highly customizable, efficient way to add dynamic side panels to your web projects. With these panels, you can enhance the overall interactivity of your website and create a more engaging user experience. By understanding the installation process, panel attributes, and configuration, you can unlock the full potential of AngularJS Panels.