Angular UI Tree is a powerful AngularJS component that allows for easy sorting of nested lists and drag-and-drop functionality without the need for jQuery. It offers native AngularJS scope data binding, making it a popular choice among developers. In this article, we will dive into the features, requirements, and usage of Angular UI Tree, as well as provide some examples to showcase its capabilities.
Features of Angular UI Tree
Angular UI Tree comes with a range of features that make it a versatile and efficient tool for managing nested lists. Let’s take a closer look at some of its key features:
Native AngularJS Scope Data Binding
One of the main advantages of using Angular UI Tree is its ability to bind data directly from the AngularJS scope. This means that any changes made to the tree structure will automatically be reflected in the underlying data model. This makes it easier to manage and manipulate data within the tree, as there is no need to manually update the data model.
Sorting and Moving Items Within the Tree
With Angular UI Tree, you can easily sort and move items within the tree structure. This is particularly useful when working with large and complex nested lists, as it allows for quick reorganization of the data. The sorting and moving functionality is also fully customizable, giving you full control over how the items are sorted and moved.
Prevention of Elements from Accepting Child Nodes
Another useful feature of Angular UI Tree is the ability to prevent certain elements from accepting child nodes. This is particularly helpful when working with hierarchical data structures, as it ensures that the tree remains organized and prevents any accidental nesting of items.
Requirements for Using Angular UI Tree
Before we dive into the usage of Angular UI Tree, let’s first take a look at the requirements for using this component in your project.
AngularJS Installation
As Angular UI Tree is an AngularJS component, you will need to have AngularJS installed in your project before you can use it. If you are not familiar with AngularJS, you can follow the official documentation for installation instructions.
Using Bower
The recommended way to install Angular UI Tree is through Bower, a popular package manager for front-end dependencies. To install Angular UI Tree using Bower, simply run the following command in your terminal:
bower install angular-ui-tree
This will automatically download and install the latest version of Angular UI Tree in your project.
Download from GitHub
Alternatively, you can also download the source code for Angular UI Tree directly from its GitHub repository. This gives you more control over which version of the component you want to use, as well as the ability to make any necessary modifications to the source code.
Usage of Angular UI Tree
Now that we have covered the features and requirements of Angular UI Tree, let’s take a look at how to use it in your project.
Load CSS
The first step is to load the CSS file for Angular UI Tree into your project. This can be done by adding the following line of code to your HTML file:
<link rel="stylesheet" href="bower_components/angular-ui-tree/angular-ui-tree.min.css">
If you have downloaded the source code from GitHub, you will need to change the path to the CSS file accordingly.
Load Script
Next, you will need to load the JavaScript file for Angular UI Tree into your project. This can be done by adding the following line of code to your HTML file:
<script src="angular-ui-tree.js"></script>
Alternatively, if you prefer to use the minified version, you can use the following code instead:
<script src="angular-ui-tree.min.js"></script>
Again, if you have downloaded the source code from GitHub, you will need to change the path to the JavaScript file accordingly.
Module Dependency
Finally, you will need to add ui.tree as a dependency to your AngularJS module. This can be done by adding the following line of code to your module definition:
angular.module('myApp', ['ui.tree']);
Once you have completed these steps, you are now ready to start using Angular UI Tree in your project.
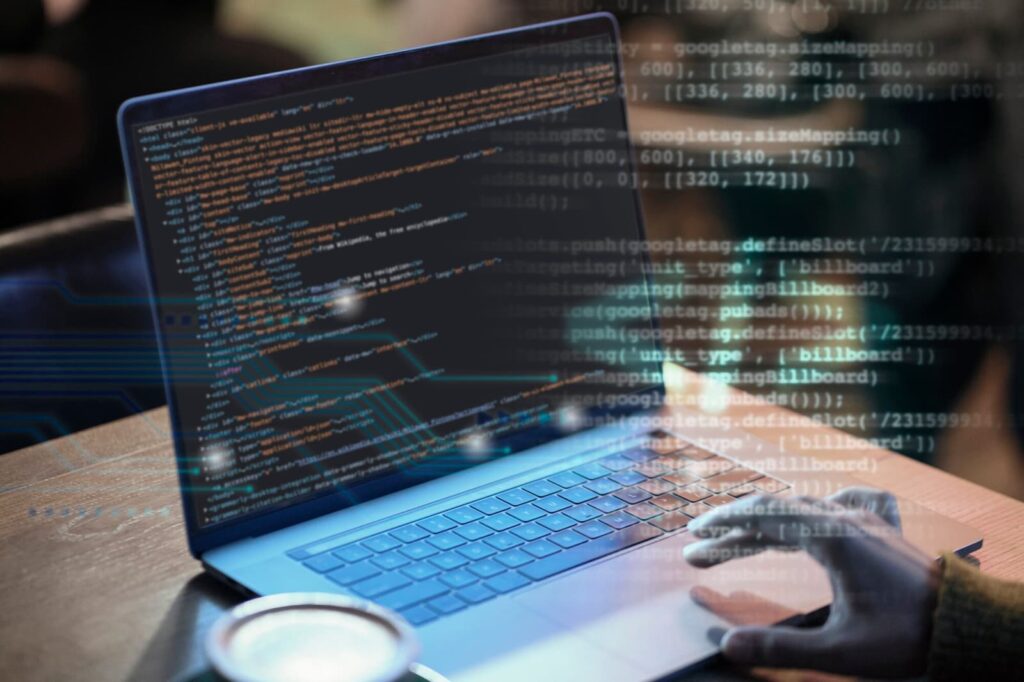
Angular UI Tree Examples
To give you a better understanding of how Angular UI Tree works and what it is capable of, let’s take a look at some examples.
Example 1: Simple Tree Structure
In this example, we will create a simple tree structure with three levels of nesting. The data for our tree will be defined in the controller as follows:
$scope.treeData = [
{
"id": 1,
"title": "Parent 1",
"nodes": [
{
"id": 11,
"title": "Child 1",
"nodes": []
},
{
"id": 12,
"title": "Child 2",
"nodes": []
}
]
},
{
"id": 2,
"title": "Parent 2",
"nodes": [
{
"id": 21,
"title": "Child 1",
"nodes": []
},
{
"id": 22,
"title": "Child 2",
"nodes": []
}
]
}
];
Next, we will use the ui-tree directive to render the tree in our HTML file:
<div ui-tree>
<ol ui-tree-nodes ng-model="treeData">
<li ng-repeat="node in treeData" ui-tree-node>
<div ui-tree-handle>{{node.title}}</div>
<ol ui-tree-nodes ng-model="node.nodes">
<li ng-repeat="child in node.nodes" ui-tree-node>
<div ui-tree-handle>{{child.title}}</div>
</li>
</ol>
</li>
</ol>
</div>
This will result in a simple tree structure with two parent nodes and two child nodes under each parent. The ui-tree directive is used to initialize the tree, while the ui-tree-nodesdirective is used to define the nodes of the tree. The ng-model attribute is used to bind the data from the controller to the tree.
Example 2: Customizing Sorting and Moving Functionality
In this example, we will customize the sorting and moving functionality of Angular UI Tree. We will use the same data structure as in the previous example, but this time we will add some additional options to the ui-tree directive:
<div ui-tree="options">
<!-- tree structure -->
</div>
In our controller, we will define the options object as follows:
$scope.options = {
dragMove: function (event) {
// custom logic for moving items
},
accept: function (sourceNodeScope, destNodesScope, destIndex) {
// custom logic for accepting child nodes
}
};
The dragMove option allows us to define custom logic for moving items within the tree. Similarly, the accept option allows us to define custom logic for accepting child nodes. This gives us more control over how the sorting and moving functionality works in our tree.
Example 3: Preventing Elements from Accepting Child Nodes
In this example, we will use the accept option to prevent certain elements from accepting child nodes. Let’s say we want to prevent the parent nodes from accepting any child nodes. We can achieve this by adding the following code to our accept function:
$scope.options = {
// other options
accept: function (sourceNodeScope, destNodesScope, destIndex) {
if (destNodesScope.$element.hasClass('parent-node')) {
return false;
}
return true;
}
};
Here, we are checking if the destination node has the class parent-node, and if it does, we are preventing it from accepting any child nodes. This ensures that our tree remains organized and prevents any accidental nesting of items.
Conclusion
Angular UI Tree is a powerful AngularJS component that offers a range of features for managing nested lists. Its native AngularJS scope data binding, sorting and moving functionality, and prevention of elements from accepting child nodes make it a popular choice among developers. In this article, we have covered its features, requirements, and usage, as well as provided some examples to showcase its capabilities. With this knowledge, you can now start using Angular UI Tree in your projects and take advantage of its powerful features.