Integrating a datetime picker into an Angular application is an essential skill for modern web developers. With the power of Angular combined with the sleek design of Bootstrap, crafting an elegant and user-friendly date and time selection interface is easier than ever. This article dives into the Angular Datetime Picker powered by Bootstrap, guiding you through its setup, customization, and advanced features. Perfect for both beginners and seasoned developers, this guide promises to enhance your web development toolkit.
What is Angular Datetime Picker?
Angular Datetime Picker is a directive that allows users to select a date and time through an easy-to-use interface. It’s built to work seamlessly with Angular and Bootstrap, providing a responsive and intuitive UI component. This tool simplifies date and time input, making it a breeze for users to interact with forms and data on your Angular-based web applications.
Setting Up the Angular Datetime Picker
To get started with Angular Datetime Picker, ensure you have the necessary packages installed. Follow these steps:
Install the Necessary Packages
Begin by installing Bootstrap and the datetime picker module in your Angular project using npm:
npm install bootstrap angular-datetime-picker |
This command installs both Bootstrap for styling and the Angular Datetime Picker module.
Import the Module
In your Angular module file (e.g., app.module.ts), import the DatetimePickerModule:
import { DatetimePickerModule } from ‘angular-datetime-picker’; |
This step ensures that Angular recognizes and can utilize the datetime picker module throughout your application.
Add Bootstrap Styles
To apply Bootstrap’s CSS styling to your Angular project, include Bootstrap’s CSS file. This step ensures consistent styling across your application:
<link rel=”stylesheet” href=”path/to/bootstrap.css”> |
Ensure that the path to Bootstrap’s CSS file is correctly specified.
Use the Directive
Now that you’ve installed the necessary packages and imported the datetime picker module, you can easily implement the Angular Datetime Picker in your templates. Utilize the provided directive as follows:
<datetime-picker [(ngModel)]=”yourModel”></datetime-picker> |
Replace “yourModel” with the variable in your component that will store the selected date and time.
Customizing the Angular Datetime Picker
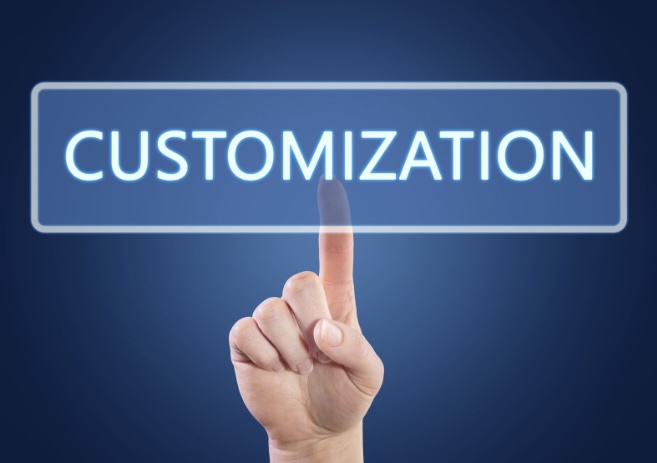
Angular Datetime Picker is a versatile tool that allows developers to display and select dates and times conveniently. Here’s how you can customize it effectively:
CSS Customization
CSS customization is one of the most straightforward ways to alter the appearance of the Angular Datetime Picker. By leveraging Bootstrap’s CSS or writing custom styles, developers can achieve a personalized look that aligns with their project’s design requirements. Below is a breakdown of how you can use CSS to customize different aspects of the Angular Datetime Picker:
Aspect | CSS Customization |
---|---|
Date and Time Display | Adjust font size, color, and alignment. |
Calendar Icon | Modify the size, color, and position of the calendar icon. |
Dropdowns | Customize the appearance of dropdown menus for selecting dates and times. |
Borders and Shadows | Add borders, shadows, or other visual effects to enhance the picker’s appearance. |
Hover Effects | Implement hover effects to provide visual feedback when users interact with the picker. |
Configuration Options
The Angular Datetime Picker offers a range of configuration options that allow developers to fine-tune its behavior and appearance. These options include:
- Date Formats: Specify the format in which dates should be displayed within the picker. For example, developers can choose between formats like MM/DD/YYYY, YYYY-MM-DD, or custom formats tailored to their needs;
- Default Values: Set default values for the date and time picker. This is particularly useful when pre-populating the picker with specific dates or times upon initialization;
- Min/Max Date Settings: Define minimum and maximum date ranges that users can select within the picker. This ensures that users cannot choose dates outside of the specified range, which is crucial for maintaining data integrity and preventing errors.
By configuring these options, developers can create a seamless and intuitive user experience with the Angular Datetime Picker.
Advanced Features
When implementing Angular Datetime Picker in your projects, it’s crucial to leverage its advanced features to enhance user experience and simplify complex tasks. Let’s delve into two key advanced features: handling time zones and responsive design.
Handling Time Zones
Dealing with time zones can introduce complexities into your application, especially when users are spread across different regions. Angular Datetime Picker offers robust functionality to simplify timezone management.
Feature | Description |
---|---|
Time Zone Offset | Allow users to specify their time zone offset, ensuring that dates and times are displayed accurately for their region. |
UTC Conversion | Automatically convert dates and times to Coordinated Universal Time (UTC) to maintain consistency across time zones. |
Time Zone Labels | Display time zone labels alongside date and time selections, providing clarity and avoiding confusion for users. |
By utilizing these settings, developers can ensure that users across different time zones have a consistent and seamless experience when interacting with the Angular Datetime Picker.
Responsive Design
With the prevalence of mobile devices and tablets, responsive design is crucial for providing a seamless user experience across various screen sizes. Angular Datetime Picker integrates seamlessly with Bootstrap, making it inherently responsive.
- Fluid Grid System: Bootstrap’s fluid grid system allows the Angular Datetime Picker to adapt dynamically to different screen sizes, ensuring optimal layout and usability;
- Media Queries: Bootstrap incorporates media queries, enabling the Angular Datetime Picker to apply specific styles and adjustments based on the device’s screen size and orientation;
- Mobile-First Approach: Bootstrap follows a mobile-first approach, prioritizing the design and functionality for smaller screens, which enhances the user experience on mobile devices and ensures compatibility across all devices.
By leveraging Bootstrap’s responsive design features, developers can ensure that the Angular Datetime Picker delivers a consistent and intuitive user experience across desktops, laptops, tablets, and smartphones.
Integration Tips
Integrating Angular Datetime Picker seamlessly into your Angular applications involves working with forms and interacting with backend services effectively. Let’s explore some integration tips for maximizing the functionality and usability of Angular Datetime Picker.
Working with Forms
Integrating Angular Datetime Picker with Angular forms is essential for capturing and managing date and time inputs efficiently. Whether you’re using template-driven or reactive forms, Angular Datetime Picker offers seamless integration options.
Form Type | Integration Approach |
---|---|
Template-Driven | Utilize Angular Datetime Picker directives within template-driven forms, binding them to form controls for easy data manipulation. |
Reactive Forms | Incorporate Angular Datetime Picker components into reactive forms, integrating them with form controls and validators as needed. |
By leveraging these integration methods, developers can ensure that Angular Datetime Picker seamlessly interacts with Angular forms, enabling users to input and manipulate date and time data effortlessly.
Interacting with Backend Services
When communicating with backend services, formatting date and time data correctly is crucial to ensure compatibility and accuracy. Angular Datetime Picker simplifies this process by providing convenient methods for formatting date and time data before sending it to the server.
- Date Formatting: Utilize Angular Datetime Picker’s built-in functions to format dates according to your server’s requirements, ensuring consistency and compatibility;
- Time Formatting: Apply appropriate formatting to time data, including time zones if necessary, to ensure accurate representation and interpretation on the server side;
- Data Transmission: Implement data transmission protocols that handle date and time data seamlessly, such as JSON or XML serialization/deserialization, to facilitate smooth communication between the frontend and backend.
By following these best practices for interacting with backend services, developers can ensure that date and time data processed by Angular Datetime Picker is formatted correctly, minimizing potential errors and ensuring smooth data transmission.
Conclusion
The Angular Datetime Picker is an incredibly useful tool for Angular developers. Its integration with Bootstrap makes it not only functional but also aesthetically pleasing. Whether you’re building complex enterprise applications or simple web projects, this datetime picker is an indispensable component. Beyond this, for those seeking advanced data visualization, Angular Google Charts offers a comprehensive suite of charting tools that can enhance your applications. With its wide range of chart types and easy integration with Angular applications, Angular Google Charts provides a dynamic solution for presenting complex data in an accessible and visually appealing way.
As you deepen your expertise with these tools, remember that practice is key. Don’t hesitate to experiment with different settings and customization options, thereby unlocking the full potential of Angular in your development projects.
FAQ
Yes, you can set minimum and maximum date options to restrict the date range.
Angular Datetime Picker is compatible with most Angular versions. Always check the documentation for the latest compatibility information.
Use the appropriate time zone settings within Angular Datetime Picker to manage different time zones.
While it’s designed to work best with Bootstrap, you can customize the styles to use it without Bootstrap’s CSS.
Absolutely! The responsiveness provided by Bootstrap ensures a smooth experience on mobile devices.