Drawing inspiration from the CleverStack Angular framework, this AngularJS Project Foundation offers a streamlined workflow for developers looking to leverage a modern AngularJS development environment. Crafted for rapid, test-driven development, this tool ensures that essential features and commands are at your fingertips. Should you encounter any missing functionality, contributions through issue reporting or pull requests are highly encouraged.
You may also like our article on the Angular Image Cropper, which shows how to apply Angular Seed’s development principles to incorporate image cropping functionality into your AngularJS projects.
Front-End Development Workflow Features
This front-end development workflow offers an extensive suite of features designed to enhance productivity and efficiency across various stages of development:
- Development Environment Enhancements: It features a development server equipped with Live Reload, facilitating real-time updates and immediate feedback on changes. This server is enhanced with jsHint for JavaScript code quality checking and LESS for dynamic stylesheet generation;
- Automated Testing Frameworks: The workflow includes a unit testing server that supports fully automated tests using Karma and Jasmine, allowing for thorough examination of individual units of code. For end-to-end (E2E) testing, it provides a server capable of running comprehensive tests through Selenium WebDriver and Protractor, ensuring that the application behaves as expected from the user’s perspective. Additionally, browserless testing is possible through PhantomJS, enabling tests to run without the need for a graphical web browser interface;
- Quality Assurance Tools: To maintain high-quality code standards, the workflow generates code coverage reports. These reports help developers identify untested parts of the codebase, ensuring thorough testing coverage. For API documentation, it integrates an API documentation server specifically for AngularJS, utilizing Docular for generating easily navigable documentation;
- Preview and Production Tools: A production preview server is included to facilitate quick reviews of the latest production builds, streamlining the process of preparing for deployment.
On the technology front, this workflow incorporates several key web technologies to streamline development and management processes:
Tool | Purpose |
---|---|
Grunt | Utilized for automating repetitive tasks such as minification, compilation, unit testing, linting, etc. |
Bower | A package management system for managing JavaScript dependencies, ensuring accessibility and updates. |
Node/NPM | Serves as the backbone for managing JavaScript packages, providing a vast repository of libraries and tools for development. |
Included Out-of-the-Box Enhancements:
- Bootstrap 3: This workflow incorporates Bootstrap 3, the most popular HTML, CSS, and JS framework for developing responsive, mobile-first projects on the web;
- COMPASS: A comprehensive CSS framework that extends CSS with dynamic behavior such as variables, mixins, and functions for more efficient styling.
Setting Up Your Angular Application
Embarking on the setup of your Angular application is streamlined with a series of commands designed to clone, configure, and launch your project environment. Follow these steps to get your Angular app up and running:
Begin by cloning the repository to your desired location with the command:
git clone https://github.com/clevertech/cleverstack-angular-seed.git my-angular-app
This action copies the Angular seed project into a new directory named my-angular-app.
Navigate into your newly created project directory:
cd my-angular-app
This step takes you into the root of your Angular application.
Install the necessary dependencies and prepare your project for launch:
grunt install
This command leverages Grunt to automatically install all required dependencies, ensuring your project is set up with all the tools it needs.
Finally, initiate your development server:
grunt server
By executing this command, you launch a local development server that serves your Angular application, often with live reloading capabilities to reflect changes in real time.
Requirements for Setup
Before beginning, ensure you have NodeJS & NPM (version 0.8 or higher) installed. Execution of the $ grunt install command will install the necessary components, which include Ruby & COMPASS, Bower, and Selenium WebDriver & Protractor.
Command Overview
Execute the following commands from your preferred terminal:
- $ grunt server – Initializes development, documentation, production preview, and automatic unit testing servers. Ideal for daily development with concurrent auto testing;
- $ grunt server:dev – Launches only the development server;
- $ grunt server:test – Launches only the automatic unit testing server;
- $ grunt server:dist – Launches only the production preview server;
- $ grunt server:docs – Launches only the documentation server;
- $ grunt test – Starts the automatic unit testing server, synonymous with grunt autotest:unit;
- $ grunt test:unit – Executes a single run of the unit tests;
- $ grunt test:coverage – Generates a unit test coverage report;
- $ grunt test:e2e – Performs a single run of end-to-end (e2e) tests;
- $ grunt autotest:e2e – Initializes the automatic end-to-end (e2e) unit testing server;
- $ grunt build – Constructs a production-ready application;
- $ grunt docs – Compiles the documentation and initiates the documentation server;
- $ grunt docs:build – Compiles the API documentation.
Configuration Files Overview
Below are the configurable files within the project:
- config/application.conf.json – Contains settings for configuring the application;
- config/environment.conf.json – Contains settings for configuring your environment;
- config/spec-unit.conf.json – Contains settings for unit testing configurations;
- config/spec-e2e.conf.json – Contains settings for end-to-end (e2e) testing configurations;
- Gruntfile.js – The core Grunt task runner file. Modification requires careful understanding of its functions;
- bower.json – Used to add JavaScript library dependencies;
- package.json – Used to add NPM package dependencies.
Project Structure and Server Details
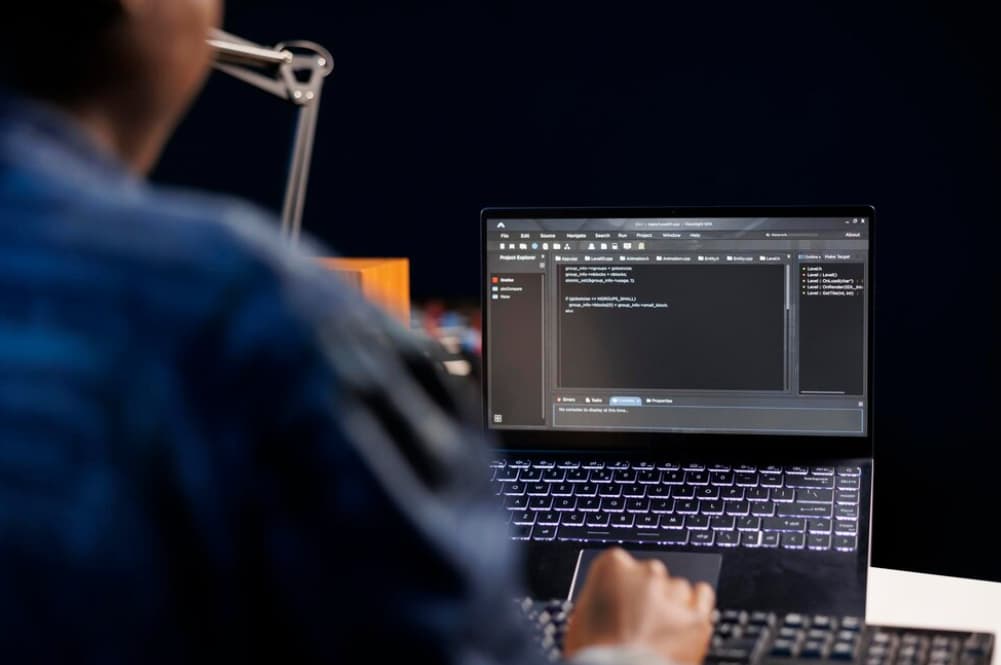
Here’s an overview of the project’s structure:
- app/ – This directory hosts the Angular app development files;
- config/ – Contains configuration files for the application;
- dist/ – Holds the most recent production build of the application;
- test/ – Includes all the test specifications for the application;
- .bowerrc – Stores Bower configuration, specifying the app path;
- .gitignore – Lists files and directories ignored by git;
- .jshintrc – Provides settings for JSHint code quality checks;
- .travis.yml – Manages Continuous Integration build status;
- api.doc – Dedicated to API documentation generation;
- bower.json – Describes JavaScript dependencies of the app;
- Gruntfile.js – Central Grunt configuration for task management;
- package.json – Lists dependencies and npm packages;
- LICENSE – Contains the license details of the app;
- README.md – Offers a comprehensive overview of the app.
Servers
- Development Server
Port: 9000
Automates the workflow for AngularJS with features like live reload, JSHint syntax checking, source map support, LESS file compilation, and autoprefixing for CSS styles. It also provides a Bootstrap 3 UI preview with live reload at /ui.html.
- API Documentation Server
Port: 9999
Serves API documentation for the AngularJS application.
- Unit Testing Server
Port: 9090
Facilitates automated unit testing using the Karma test runner.
- Unit Testing Code Coverage Server
Port: 5555
Offers code coverage reports for unit tests, accessible through port 5555.
- End-to-End Testing Server
Port: 9000
Supports end-to-end testing with Selenium WebDriver & AngularJS Protractor Framework.
- Production Preview Server
Port: 9009
Provides a glimpse into the latest production build of the application.
API Documentation Creation
Generating Your App’s API Documentation
To construct your application’s API documentation, execute the grunt docs:build command. Subsequently, access the documentation instantly by navigating to /docs on the API Documentation Server at port 9999. The documentation assembly leverages Docular.
Code Coverage Reporting
Generating Unit Test Coverage Reports
Initiate the creation of unit test coverage reports for the specified browsers in your config/spec-unit.conf.js by running grunt test:coverage. This action produces reports in the /test/coverage directory, which can be immediately viewed on the Code Coverage Server via port 5555. These reports are generated using Karma and Istanbul.
Deployment
Preparing Your Application for Production
Forge a production-ready version of your application swiftly by executing the grunt build command. This process entails handling any coding abstractions, such as CoffeeScript, asset minification, image compression, and gathering everything within the /dist directory for immediate preview on the production preview server at port 9009. The dist folder is then ready to be deployed as usual.
Development Recommendations
Modify core application Bootstrap 3 styles and scripts in the following locations:
app/bower_components/bootstrap/js/*.js
app/bower_components/bootstrap/less/*.less
Incorporate additional styles in:
app/styles/*.scss
Enforce writing unit tests for all code and keep the unit test server active for ongoing automatic testing. Ensuring your code is tested is your responsibility as a developer, offering proof of functionality.
Limit end-to-end (e2e) tests to essential scenarios only. If uncertain about what to test e2e, consult AngularJS testing strategies.
Troubleshooting
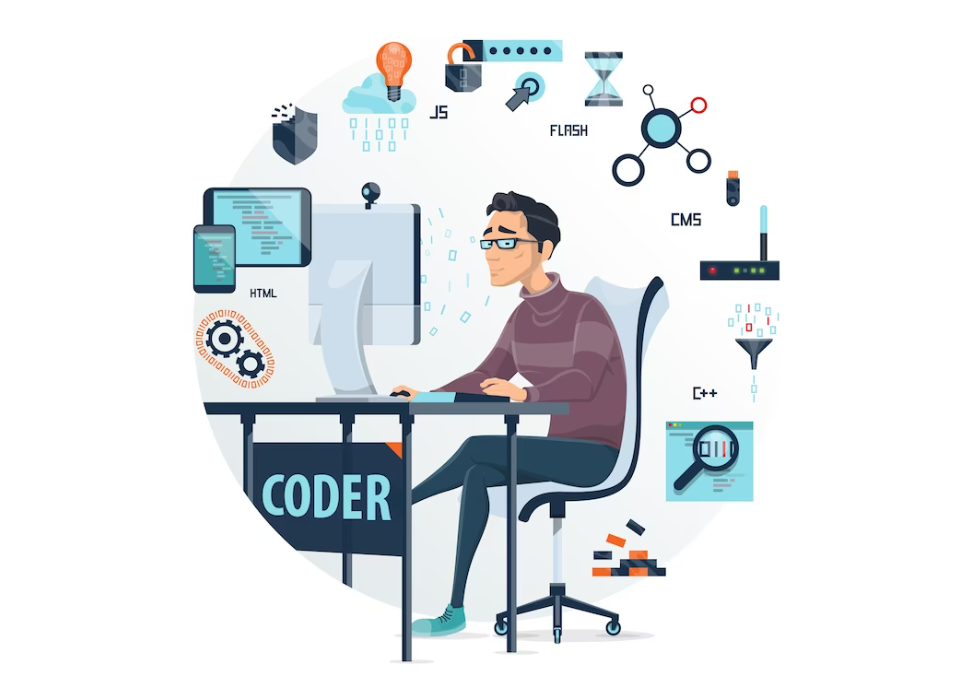
NodeJS & NPM Installation Issues
For challenges installing NodeJS, consider these resources:
Bower Installation
Install Bower via NPM with:
$ npm install -g bower
Then, manage your dependencies:
$ bower install
Dependencies install to a directory specified in .bowerrc, which is advisable not to alter to prevent issues with your development server and build process.
Ruby & COMPASS Installation
For COMPASS, using RVM is the simplest installation method:
$ curl -L https://get.rvm.io | bash -s stable --rails --autolibs=enabled --ruby=1.9.3
$ . ~/.profile
$ source /etc/profile.d/rvm.sh
$ rvm install 2.0.0
$ rvm use 2.0.0
$ ruby -v
On Linux/Debian, install Ruby with:
$ sudo apt-get install ruby
$ ruby -v
Windows users can opt for the RubyInstaller: RubyInstaller project
Install COMPASS via Ruby Gem:
$ gem update --system
$ gem install compass
Selenium, WebDriver & Protractor Installation
For seamless installation, use the Protractor NPM package for Selenium Server:
$ npm install -g protractor
Adjust the Chrome Driver path in config/spec-e2e.conf.json based on your operating system. If using Selenium Server separately (optional), start it with:
$ cd <project dir>
$ webdriver-manager start
Initiate automated e2e testing with:
$ grunt autotest:e2e
Alternatively, manually download the latest Selenium Server .jar file and Chrome Driver, placing them in /test/selenium/ and update config/spec-e2e.conf.json accordingly.
Frequently Asked Questions
Grunt Usage
To introduce new Grunt tasks, modify the Gruntfile.js. For ease of development, you can incorporate options in the config/*.conf.js files.
To introduce new Grunt tasks, modify the Gruntfile.js. For ease of development, you can incorporate options in the config/*.conf.js files.
Yes, Grunt can be configured to support HTTPS. You’ll need to adjust the server options in the Gruntfile.js.
Unit & End-to-End (E2E) Testing
The E2E test page displays the Selenium Server Hub, which requires the webdriver manager to be running in a separate console for operation. Selenium WebDriver and Protractor E2E testing are areas of active development and improvements.
In the browser where the test is captured, click the “DEBUG” button or navigate to http://localhost:9876/debug.html to debug using the web inspector. Refresh the debug page if necessary once the inspector is open.
Karma supports various testing frameworks like QUnit, Jasmine, and Mocha/Chai without needing additional plugins. While Jasmine is the default, Karma is flexible with framework choice.
The choice of Mocha over others like Jasmine or QUnit was based on preference, aiming for consistency in testing both front-end (AngularJS) and back-end (NodeJS) aspects. Mocha’s growing adoption in new projects was also a factor. The decision was supported by community input and endorsements from figures like Paul Irish.
Karma is designed for unit testing, focusing on testing the logic within controllers, directives, and services. Protractor is better suited for end-to-end or integration testing, simulating a user’s interaction with the application. Tests mimicking user instructions are ideal for Protractor. This distinction aligns with the guidance from Protractor Docs FAQ.
Bootstrap 3 & LESS
Yes, it supports both CSS and LESS, with an autoprofixer to handle legacy browser prefixes automatically.
To keep changes to Bootstrap source files through version control, you can remove the corresponding line from the .gitignore file, allowing your modifications to persist.
The preference for LESS was primarily due to its independence from Ruby, unlike SASS which historically required Ruby. Although there are non-Ruby versions of SASS (libsass), they lacked features like sourcemaps support and were under development at the time of decision.
Bower
Currently, it is required. Plans are underway to potentially remove this requirement to streamline the dist directory.
Miscellaneous
editorconfig helps maintain consistent coding styles for all developers on a project, ensuring uniformity across various editors and IDEs.
Conclusion
Angular Seed, influenced by the CleverStack Angular framework, is a practical tool for developers starting with AngularJS. It focuses on efficient and test-driven development, offering a simplified workflow and essential features for quick project setup. The project encourages community contributions, such as reporting issues or submitting pull requests, to improve its functionality. Angular Seed aims to help developers build robust and maintainable web applications more efficiently.