Efficient management of dates and date ranges holds significant importance in contemporary web development, particularly within applications focused on scheduling, analytics, or data visualization. Angular, a widely utilized JavaScript framework, presents an array of utilities and libraries aimed at optimizing this aspect. Among these resources stands the Angular DateRangePicker, designed to facilitate seamless selection of date ranges for end-users. Within the following discourse, we shall explore the Angular DateRangePicker comprehensively, encompassing its functionalities, integration methodologies, and avenues for customization.
Exploring Angular DateRangePicker:
The Angular DateRangePicker stands as an essential UI component within web applications, revolutionizing the way users interact with date selection. Seamlessly integrated into Angular frameworks, this tool offers an intuitive interface, empowering users to effortlessly select precise time intervals. Let’s delve deeper into its functionalities and benefits.
Key Features:
- Date Range Selection:
- Empowers users to effortlessly select date ranges by interacting with the visually appealing calendar interface;
- Enables precise selection by simply clicking on the desired start and end dates within the DateRangePicker.
- Predefined Ranges:
- Simplifies the selection process with a myriad of predefined date ranges such as ‘Today’, ‘Yesterday’, ‘Last 7 days’, ‘Last 30 days’, and more;
- Enhances user efficiency by offering quick access to commonly used time intervals, streamlining workflows.
- Customization Options:
- Offers developers unparalleled flexibility in tailoring the DateRangePicker to match application-specific requirements;
- Allows customization of appearance and behavior, including date formats, disabled dates, minimum and maximum selectable dates, and more;
- Facilitates seamless integration into diverse projects, ensuring a cohesive user experience across applications.
- Localization Support:
- Recognizes the global nature of web applications by providing robust localization support;
- Enables developers to display date formats and labels in various languages and regions, catering to a diverse user base;
- Enhances accessibility and usability for international audiences, fostering inclusivity within applications.
Benefits and Recommendations:
- Improved User Experience:
- Enhances user satisfaction by simplifying the date selection process, reducing cognitive load, and improving efficiency;
- Facilitates seamless navigation and interaction, fostering a positive user experience across web applications.
- Efficiency and Productivity:
- Streamlines workflows by offering quick access to predefined date ranges, minimizing manual input and accelerating task completion;
- Empowers users to focus on core tasks by eliminating the complexities associated with date selection, enhancing productivity.
- Enhanced Customization:
- Tailor the DateRangePicker to match the visual aesthetics and functional requirements of your application, ensuring seamless integration and alignment with branding guidelines;
- Leverage customization options to adapt the DateRangePicker to unique use cases, optimizing user engagement and satisfaction.
- Global Accessibility:
- Utilize localization support to cater to diverse audiences across different regions and languages, expanding the reach of your application;
- Enhance inclusivity by providing a localized experience, fostering engagement and resonance with international users.
Angular DateRangePicker Implementation Guide
Integrating a DateRangePicker into your Angular application can significantly enhance its functionality, allowing users to conveniently select date ranges. Here’s a comprehensive guide on implementing the Angular DateRangePicker seamlessly into your project:
Step 1: Install Dependencies
Before diving into implementation, ensure you have the necessary dependencies installed. Follow these steps:
Open your terminal. Run the following command to install the required package:
npm install @syncfusion/ej2-angular-calendars
Step 2: Import DateRangePicker Module
Once you’ve installed the dependencies, it’s time to import the DateRangePicker module into your Angular application. Here’s how:
Navigate to the Angular module where you want to use the DateRangePicker. Import the DateRangePickerModule from the ‘@syncfusion/ej2-angular-calendars’ package. Add it to the imports array within the NgModule decorator.
import { DateRangePickerModule } from '@syncfusion/ej2-angular-calendars';
@NgModule({
imports: [
// Other modules
DateRangePickerModule
],
// Other configurations
})
export class AppModule { }
Step 3: Add DateRangePicker Component
Now that you’ve imported the module, you can effortlessly add the DateRangePicker component to your Angular template. Here’s how:
Open the template file where you want to place the DateRangePicker. Use the <ejs-daterangepicker></ejs-daterangepicker> tag to include the DateRangePicker component.
<ejs-daterangepicker></ejs-daterangepicker>
Step 4: Customize as Needed
One of the key advantages of using the Angular DateRangePicker is its flexibility and customization options. You can tailor it to suit your specific requirements by leveraging its properties and events. Here are some customization tips:
- Set Start and End Dates: Define the default start and end dates to guide users;
- Apply Date Formatting: Customize the date format to match your application’s design;
- Enable/Disable Specific Dates: Control which dates users can select based on your application’s logic;
- Implement Event Handlers: Utilize events like change or select to trigger actions based on user interactions;
- Localization: Adapt the DateRangePicker to different languages and regions for a global audience.
Enhanced Date Range Picker Component with Angular
If you’re looking to integrate a dynamic and user-friendly date range picker component into your Angular application, you’re in the right place. In this guide, we’ll walk you through setting up and customizing the EJS Date Range Picker component with Angular, empowering you to effortlessly manage date selections within your web app.
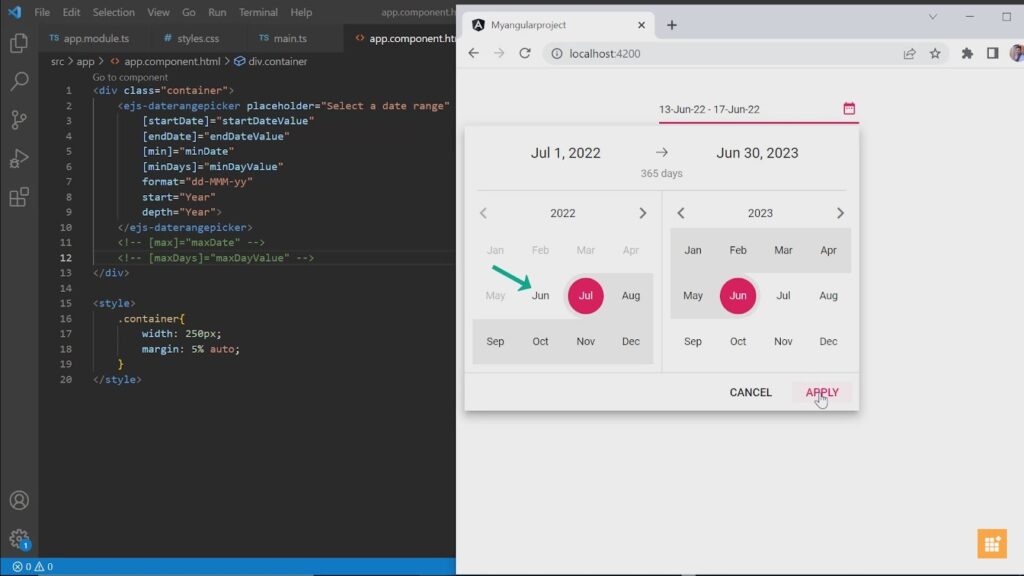
Getting Started
Before diving into implementation, ensure you have the necessary prerequisites installed:
- Node.js and npm: Ensure you have Node.js and npm (Node Package Manager) installed on your system. You can download and install them from the official Node.js website;
- Angular CLI: Install the Angular CLI globally using npm. This command will provide you with the necessary tools for Angular development.
npm install -g @angular/cli
Integrating the Date Range Picker Component
Once your environment is set up, follow these steps to integrate the EJS Date Range Picker component into your Angular project:
Install Essential Packages: Begin by installing the required EJS packages via npm. Run the following command in your terminal:
npm install @syncfusion/ej2-angular-calendars --save
Import Module: Import the DateRangePickerModule into your Angular module file (e.g., app.module.ts) to make it available throughout your application.
typescript
Copy code
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { DateRangePickerModule } from '@syncfusion/ej2-angular-calendars';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
DateRangePickerModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Usage in Component: Now, you can use the EJS Date Range Picker component within your Angular components. For instance, in your app.component.ts file:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
startDate: Date = new Date('2024-01-01');
endDate: Date = new Date('2024-01-31');
placeholder: string = 'Select a date range';
dateRangeChanged(args: any): void {
// Handle date range change event
console.log(args.value);
}
}
Customization and Configuration
The EJS Date Range Picker component offers extensive customization options to tailor its appearance and behavior according to your application’s requirements. Here are some key configurations you can apply:
- Placeholder Text: Set a custom placeholder text to provide guidance to users regarding the expected input;
- Date Format: Define the format in which dates should be displayed within the picker;
- Minimum and Maximum Dates: Restrict date selection by specifying minimum and maximum allowable dates;
- Localization: Customize the component to display date formats and language specific to your target audience.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
startDate: Date = new Date('2024-01-01');
endDate: Date = new Date('2024-01-31');
placeholder: string = 'Select a date range';
dateFormat: string = 'MM/dd/yyyy'; // Customize date format
// Define minimum and maximum selectable dates
minDate: Date = new Date('2024-01-01');
maxDate: Date = new Date('2024-12-31');
dateRangeChanged(args: any): void {
// Handle date range change event
console.log(args.value);
}
}
Conclusion
The Angular DateRangePicker streamlines the selection of date ranges within Angular applications, offering an interface that is both intuitive and user-friendly. With its array of predefined ranges and extensive customization capabilities, it serves as a pivotal asset in augmenting the overall user experience. This article delineates a step-by-step approach for developers to seamlessly incorporate and tailor the DateRangePicker according to their application’s unique needs. Whether the objective is scheduling, analytics, or any other scenario necessitating date management, the Angular DateRangePicker emerges as a flexible and effective solution, poised to address diverse requirements with ease.