If you’re a developer working with AngularJS, you know the importance of creating reusable and interoperable components. This not only saves time and effort but also ensures consistency and scalability in your projects. One such component that can greatly enhance your user experience is a timer. And with the help of the Angular-Timer directive, you can easily add a timer to your AngularJS applications without any hassle. In this article, we’ll take a deep dive into the Angular-Timer directive and see how it can be used to create dynamic and interactive timers in your projects.
What is Angular-Timer?
Angular-Timer is an open-source AngularJS directive that allows you to add a timer to your web applications. It is built on top of the AngularJS framework and provides a simple and intuitive way to create timers that can be used for various purposes such as countdowns, stopwatches, or any other time-based functionality. The directive is highly customizable and can be easily integrated into your existing AngularJS projects.
Features of Angular-Timer
Before we dive into the technical details, let’s take a look at some of the key features of Angular-Timer:
- Easy to use: Angular-Timer comes with a simple and intuitive API that makes it easy to use even for beginners;
- Customizable: The directive offers a wide range of customization options, allowing you to create timers that match your project’s design and requirements;
- Interoperable: Angular-Timer is designed to work seamlessly with other AngularJS components, making it easy to integrate into your projects;
- Lightweight: The directive has a small footprint and does not add any unnecessary bloat to your application;
- Cross-browser compatible: Angular-Timer works across all modern browsers, ensuring a consistent experience for your users.
Now that we have a basic understanding of what Angular-Timer is, let’s explore its capabilities in more detail.
Getting Started with Angular-Timer
To use Angular-Timer in your project, you first need to install it using either npm or bower. Once installed, you can simply add the directive as a dependency in your AngularJS module and start using it in your HTML templates. Let’s take a look at how this is done:
Installation
To install Angular-Timer using npm, run the following command in your terminal:
npm install angular-timer --save
If you prefer using bower, you can install the directive by running the following command:
bower install angular-timer --save
Adding Angular-Timer to Your Project
Once the directive is installed, you can add it as a dependency in your AngularJS module like this:
angular.module('myApp', ['timer']);
This will make the timer directive available for use in your HTML templates.
Using Angular-Timer in Your Templates
To use Angular-Timer in your templates, simply add the timer attribute to any element where you want the timer to be displayed. You can also specify various attributes to customize the behavior of the timer. For example:
<div timer countdown="10" interval="1000" finish-callback="onFinish()">
{{seconds}}
</div>
In the above code, we have added the timer attribute to a div element and specified the countdown attribute to set the initial value of the timer to 10 seconds. We have also set the interval attribute to 1000 milliseconds, which means the timer will count down every second. Finally, we have specified a finish-callback function that will be called when the timer reaches 0. In this case, we are simply updating the seconds variable in our template, but you can perform any action you want in the callback function.
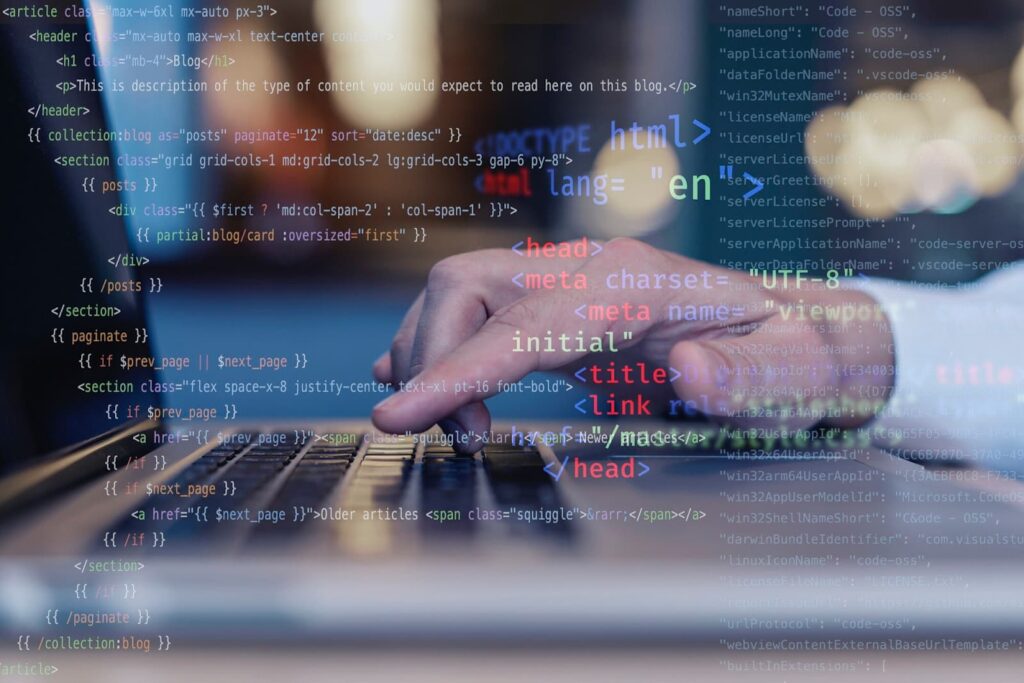
Customizing Angular-Timer
As mentioned earlier, Angular-Timer offers a wide range of customization options to suit your project’s needs. Let’s take a look at some of the key attributes that can be used to customize the behavior of the timer.
Countdown vs Stopwatch
By default, Angular-Timer works as a countdown timer, but you can also use it as a stopwatch by setting the countdown attribute to false. This will make the timer count up instead of down.
Interval
The interval attribute allows you to specify the frequency at which the timer should update. The default value is 1000 milliseconds (1 second), but you can change it to any value you want.
Finish Callback
As we saw earlier, the finish-callback attribute allows you to specify a function that will be called when the timer reaches 0. This can be useful if you want to perform any action after the timer finishes, such as redirecting the user to another page or displaying a message.
Auto Start
By default, Angular-Timer starts automatically when the page loads. However, you can disable this behavior by setting the auto-start attribute to false. This can be useful if you want to start the timer manually using a button or some other event.
Pause and Resume
Angular-Timer also provides the ability to pause and resume the timer. You can do this by calling the pause() and resume() functions respectively on the timer object in your controller. This can be useful if you want to give the user more control over the timer.
Advanced Usage
Apart from the basic functionality, Angular-Timer also offers some advanced features that can come in handy in certain scenarios. Let’s take a look at some of these features.
Multiple Timers
In some cases, you may want to have multiple timers on the same page. Angular-Timer makes this possible by allowing you to specify a unique id for each timer. This can be done by setting the id attribute on the element where the timer directive is used. For example:
<div id="timer1" timer countdown="10">
{{seconds}}
</div>
<div id="timer2" timer countdown="20">
{{seconds}}
</div>
Pausing and Resuming Individual Timers
If you have multiple timers on the same page, you may want to pause and resume them individually. This can be achieved by using the pause() and resume() functions on the timer object, as we saw earlier. However, you need to make sure that you are calling these functions on the correct timer object. To do this, you can use the getTimer() function, which returns the timer object associated with a particular id. For example:
var timer1 = $scope.getTimer('timer1');
var timer2 = $scope.getTimer('timer2');
// Pause timer1
timer1.pause();
// Resume timer2
timer2.resume();
Conclusion
In this article, we explored the Angular-Timer directive and saw how it can be used to create dynamic and interactive timers in your AngularJS projects. We covered its features, installation process, usage, and customization options. We also looked at some advanced features and answered some frequently asked questions. With Angular-Timer, you can easily add timers to your projects without having to worry about compatibility or complexity. So go ahead and give it a try in your next project!